findme Display: None vs. Visibility: Hidden
We can hide elements by declaring a display: none
value. Another way is to declare visibility: hidden
instead of display: none
, but there is a difference between them.
To show the difference, let’s hide one of the boxes below:
First I'm hiding the blue box (#box-2
) with display: none
#box-2 {
display: none;
width: 100px;
height: 100px;
background: blue;
}
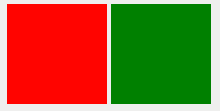
Our blue box is now removed from the view. It actually still exists on the HTML structure, but with display: none
an element behaves like it is completely deleted. As a result, the green box takes the empty place and moves to the left automatically.
However, visibility: hidden
doesn’t remove an element completely. It just makes the element invisible:
#box-2 {
width: 100px;
height: 100px;
background: blue;
visibility: hidden;
}

Block vs. Inline
Have you ever noticed that some HTML tags like <div>
, <p>
, <ul>
take full-width of space and each starts with a new line, whereas other HTML tags like <span>
, <img>
or <a>
don’t need a new line and can be placed side by side?
This is because of the different display
behaviors: Block or inline. Let’s see the difference with a short example. Without any CSS, I create an HTML template with <p>
and <span>
tags:
<body>
<p>I'm a paragraph</p>
<p>I'm a paragraph too</p>
<span>I'm a word</span>
<span>I'm a word too.</span>
</body>

Can you see the difference? Each <p>
tag starts with a new line even if there is enough space. Span
’s, however, displayed side by side.
Every HTML element has a default display value. — W3
By default, HTML elements have a display behavior as block or inline. Elements that each start with a new line (<p>
tags in this example) are called block-level elements, and the others (<span>
) which can be placed side by side are inline elements.
There are some different characteristics between block and inline elements:
Block-level elements
- Take full-width (100% width) by default
- Each gets displayed in a new line
- Width & height properties can be set
- Can contain other block or inline elements
Since <p>
tags are block-level elements, width & height properties can be set:
p {
height: 100px;
width: 100px;
background: red;
color: white;
}
If no width was declared here, then the default width of <p>
would be 100%. However, I declared a 100px width and the next <p>
element still starts with a new line:
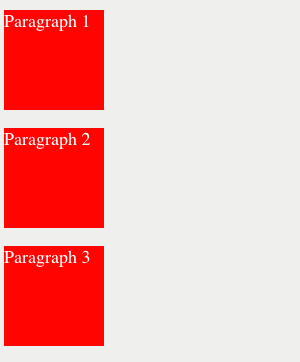
Inline elements
- Take only as much space as they need
- Displayed side by side
- Don’t accept width or height properties, and top-bottom margin
- Can be a parent of other inline elements
We can change the display behavior of elements. So let’s change the display behavior of <p>
tag to inline
:
p {
height: 100px;
width: 100px;
background: red;
color: white;
display: inline;
}
Since our <p>
tag is now an inline element, they will be placed side by side and the width & height properties have no effect anymore:

View a complete list of HTML tags as block & inline elements.
Display: Inline-block
In some cases, both of the display values may not be enough for better web design. At that point, a third display behavior comes to the rescue and also makes alignment much easier: display: inline-block
.
As we can understand from its name, display: inline-block
declaration shows both the characteristics of inline and block-level elements.
In other words, we can think of an inline element, that width & height properties can be set, or we can think of a block-level element, that doesn’t have to start with a new line.
For a clear understanding, I’m giving our <p>
tag an inline-block behavior:
p {
display: inline-block;
height: 100px;
width: 100px;
background: red;
color: white;
}
Now they can be placed side by side, and width & height properties can also be set:

You can also watch my video about CSS Display Property below:
The display
property is being used for showing, hiding, or positioning HTML elements in our layout. Every HTML element has a default display behavior and without understanding the logic behind it, you will have difficulties while working with CSS.
There is also a newer option of the display property called flex, which we use for building Flexbox layouts, and flex also provides an easier way for positioning elements. So in this post, you’re going to learn:
- What block & inline-level elements are
- Why using inline-block is good
- The flex property as an alternative
- Flex vs Inline-Flex
- How to center things easily with Flex
Block-Level Elements
Every HTML element has a default display behavior. Some HTML elements (like <div>, <p>, <ul> etc.) have a default display behavior which is known as block-level.
Block-level means that once they’re placed in the template, they will take each the full-width of a complete row.
<div class="box blue"> 1 </div><div class="box red"> 2 </div><div class="box purple"> 3 </div><div class="box orange"> 4 </div>// STYLING
.box {
border-bottom: 2px solid black;
height: 50px;
}
So these 4 div elements (boxes) above in the example are taking 100% of the space of their rows, even if I haven’t assigned any width properties:

How to place them side by side?
Now you might think that, ok if they take 100% of the width, what happens if I assign a smaller width, let’s say 20%:
.box {
width: 20%;
}
Then, they should be placed side by side, right? Well, not really…

This is a common mistake that many people do, even if you assign a smaller width, they still keep their block-level display behavior regardless of an assigned (smaller) width or not.
So if you want to place them side by side, you need to change their display behavior, let’s say to inline:
display: inline;
Inline Elements
So what is inline?
You saw above an example of block-level elements. On the other hand, there are also some inline-level elements by default (like <span> or <a> tags), which can directly be placed side by side:

You can either use <span>’s instead of <div>’s, or you can assign an inline value to the box class:
.box {
border-bottom: 2px solid black;
width: 100px;
height: 50px;
display: inline;
}
But the downside of inline elements is that we can not apply width or height properties, they just don’t work with them. If you don’t know this information, then working with CSS can be really frustrating.
One Good Solution: Inline-Block
There is also a third option: inline-block. This property takes the benefits of both block and inline-level elements.
So if you use display inline-block:
- You will be able to apply width & height properties to elements, which we can’t do with inline elements
- You can also place those elements side by side, which we can’t do with block-level elements
.box {
display: inline-block;
}

This approach has been used for a long time in CSS for the positioning of elements or changing their display behavior.
What about Display: Flex?
There is also a newer way that you can use for alignment or for designing the layout of the webpage, which is the flex property:
display: flex;
Firstly, the important thing you should know is that “flex” is not just a property like “block”, or “inline”. It is a larger CSS module with various child properties.
I will go into more details in my upcoming posts. ( For now, you can also check out this post’s tutorial video here.)
Use Flex Only for Containers
Using the flex property requires a parent (or a container) element.
The benefits of flex can only be used under the elements of its container, but it won’t work outside of it.
<div class="container">
<div> 1 </div>
<div> 2 </div>
<div> 3 </div>
<div> 4 </div>
</div>
Now let’s assign a display flex property to the container div:
.container {
display: flex;
background: gray;
}
When we do that, all of these boxes will be automatically placed side by side even without changing their display behavior:

Besides, the container (div) still takes the full width of its row and behaves like a block-level element, but now actually it is a flex-container.
Flex vs Inline-Flex
On the other hand, if you prefer to make your flex container as an inline-level flex element, then all you need to do is to change this to inline-flex:
display: inline-flex;
So the container takes the necessary space as large as its children. To make it visible, let’s also add some padding:
.container {
display: inline-flex;
padding: 10px;
background-color: gray;
}

Centering Elements with Flex
Working with flex is really beneficial, we can even do much more. For example, we can easily center the boxes with a single property called justify-content:
.container {
display: flex;
justify-content: center;
background-color: gray;
}

So now all of the children are very easily placed in the center. The justify-content property works only for flex elements. I will keep explaining more Flex features in my upcoming posts.
HTML Exambles
The div tag is known as Division tag. The div tag is used in HTML to make divisions of content in the web page like (text, images, header, footer, navigation bar, etc). Div tag has both open(<div>) and closing (</div>) tag and it is mandatory to close the tag. The Div is the most usable tag in web development because it helps us to separate out data in the web page and we can create a particular section for particular data or function in the web pages.
- Div tag is Block level tag
- It is a generic container tag
- It is used to the group of various tags of HTML so that sections can be created and style can be applied to them.
As we know Div tag is block-level tag in this example div tag contain entire width. It will be displayed div tag each time on a new line, not on the same line.
Example 1:
Output:
As we know div tag is used for grouping HTML elements together and is to apply CSS and web layout on them Lets see below example without using div tag. we need to applying CSS for each tag (in the example using H1 H2 and two paragraphs p tags)
Example 2:
Creating Web Layout using Div Tag
The div tag is a container tag inside div tag we can put more than one HTML element and can group together and can apply CSS for them.
div tag can be used for creating a layout of web pages in the below examples shows creating a web layout
we can also create web layout using tables tag but table tags are very complex to modify the layout
The div tag is very flexible in creating web layouts and easy to modify. in below example will show grouping of HTML element using div tag and create block-wise web layout.
Example:
Using Div tag we can cover gap between heading tag and paragraph tag in this example will display three blocks web layout.
we can use CSS in any divisions using the following methods:
1. Using class:
we can use Class on that particular div either in internal CSS or external CSS
- In case of internal CSS: we need to define Class in the <head> section of HTML within <style> element.
- In case of External CSS: we need to create a separate .css file and include it in HTML code in <head> section using <link> element.
The class name should be different from other class names in other div otherwise the CSS used in one div can affect another division.
- Code:
- CSS for color class: File name color.css
In this example, we used a class to that particular Div. with name color.css which properties of div. It is a separate file which is linked by link tag in this HTML code
2. Inline CSS:
we can directly use CSS in div also this method does not require of CLASS. Div in HTML coding is used as a container tag also because it is the one that can contain all other tags.
- Code:
In this method, we applying inline CSS in the div tag. By using style attribute this style will apply to that particular div.
Difference Between Div tag and span tag
The div and span tag are two common tags when creating pages using HTML and perform different functionality on them
while div tag is a block level element and span is inline element The div tag creates a line break and by default creates a division between the text that comes after the tag as begun and until the tag ends with </div>. div tag creates separate boxes or containers for all elements inside this tag like text, images, paragraphs.
Properties | Div Tag | Span Tag |
---|---|---|
Elements Types | Block-Level | Inline |
Space/Width | Contain Whole Width Available | Takes only required Width |
Examples | Headings, Paragraph, form | Attribute, image |
Uses | Web-layout | container for soome text |
Attributes | Not required,with common css, class | Not required,with common css, class |
The span tag does not create a line break similar to a div tag, but rather allows the user to separate things from other elements around them on a page within the same line. avoiding of line break, results only that selected text to change, keeping all the other elements around them same.
Below example will display the difference between span and div tag while div tag contains whole width and span tag contain only required width and rest parts are free for another element.
Code:
Supported Browser: The browser supported by <div> tag are listed below:
- Google Chrome
- Internet Explorer
- Firefox
- Opera
- Safari
Attention reader! Don’t stop learning now. Get hold of all the important Comcompetitivepetitve Programming concepts